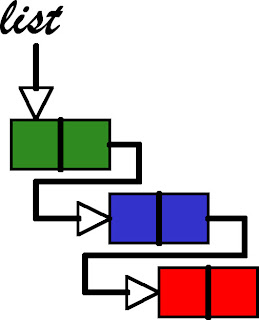
Please do not ask regarding the algorithm if your having problem translating it. Learn to read algo. If your sure something is wrong with it, call my attention.
Open your previous devC files and add this algorithm I'm about to give in Node.h.
step 1: Create a function named as Sort (lets assume you want to sort your nodes/items in ascending order) with void as its return type.
step 2: Inside your function, transform this algorithm into a C++ code.
1. Create a pointer (current) of type Node
2. Equate current to head
3. Iterate using while loop until current is null
a. Create another pointer (next) of type Node
b. Equate next to current->link
c. Iterate using a while loop until next is null
i. Test using an if statement whether current’s data value is greater compared to next’s data value (if true, proceed with the bullets list)
Ø Create a pointer (temp) of type Node
Ø Equate temp to a new Node
Ø Assign the values of current (data) to temp (data)
Ø Assign the values of next (data) to current (data)
Ø Assign values of temp (data) to next (data)
Ø Delete temp using the keyword temp
ii. move the next pointer to next->link
d. Move current pointer to current->link